Ultrasonic Range Sensor Class
The range finder class controls the ultrasonic range detectors. It works by emitting an ultrasonic sound wave and measuring the amount of time it takes for the sound to return to the sensor. It can then use the time difference to calculate distance. If a timeout event occurs then it is assumed that no object is present.
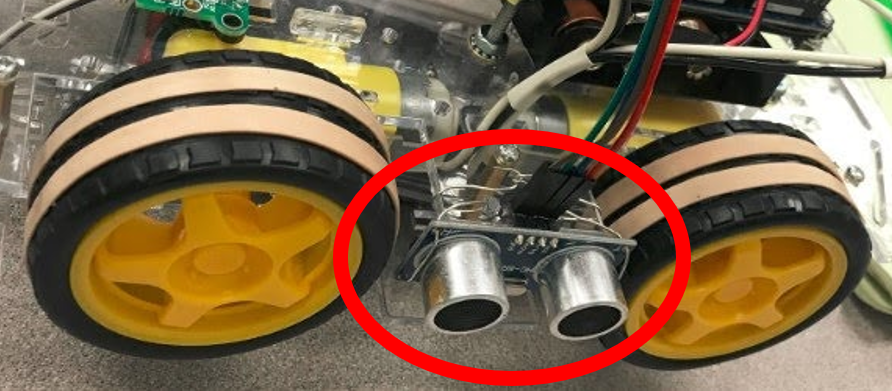
.cpp Code
#include "RangeFinder.h"
#include <arduino.h>
RangeFinder :: RangeFinder (byte Epin, byte Tpin,unsigned int SensorNum){
m_TrigTime = 0;
m_EchoTime = 0;
m_TotalTime = 0;
m_SensorNum = SensorNum;
m_Range = 0;
m_Epin = Epin;
m_Tpin = Tpin;
}
float RangeFinder :: updateRTime(float Etime){
m_TrigTime = .0006; //size of the gap between the trigger and echo
m_EchoTime =(Etime/15625.000)-m_TrigTime;
//divided by the prescaler and subtracts the difference
//or gap between the trigger and the echo pulse
return(m_EchoTime);
}
void RangeFinder :: TrigPulse() //created a pulse for the trigger{
digitalWrite(m_Tpin, LOW);
delayMicroseconds(10);
digitalWrite(m_Tpin, HIGH);
delayMicroseconds(10);
digitalWrite(m_Tpin, LOW);
delayMicroseconds(10);
}
float RangeFinder :: GetRange(){
m_Range= ((m_EchoTime*343)/2)*100; //multiplied by the speed of sound
//divided by two to get the length of the signal and multiplied by 100
//to get the results in cm.
return(m_Range);
}
.h Code
#include <arduino.h>
#ifndef RangeFinder_h
#define RangeFinder_h
class rangefinder{
private:
float m_TrigTime; // trigger time
float m_EchoTime; //echo time
float m_Range; //the distance of a wall
float m_TotalTime; //total duration time
unsigned int m_sensornumber; //the sensor that is being used
byte m_Epin;
byte m_Tpin;
public:
rangefinder(byte Epin, byte Tpin,unsigned int sensornumber); //constructor
void TrigPulse(); //generates a pulse for the trigger pin
float updateRTime(float Etime); //updates the echo duration time
float GetRange(); //gets the distance of the sensor from a wall
//bool TargetRange(); //not implemented yet
};
#endif