Maze Navigation Sketch
In order to complete the maze challenge the robot had to autonomously navigate through a maze made out of hollow cardboard boxes without touching the sides. The robot received input from the two ultrasonic range detectors and manipulated each DC motor as needed in order to turn the robot left or right.
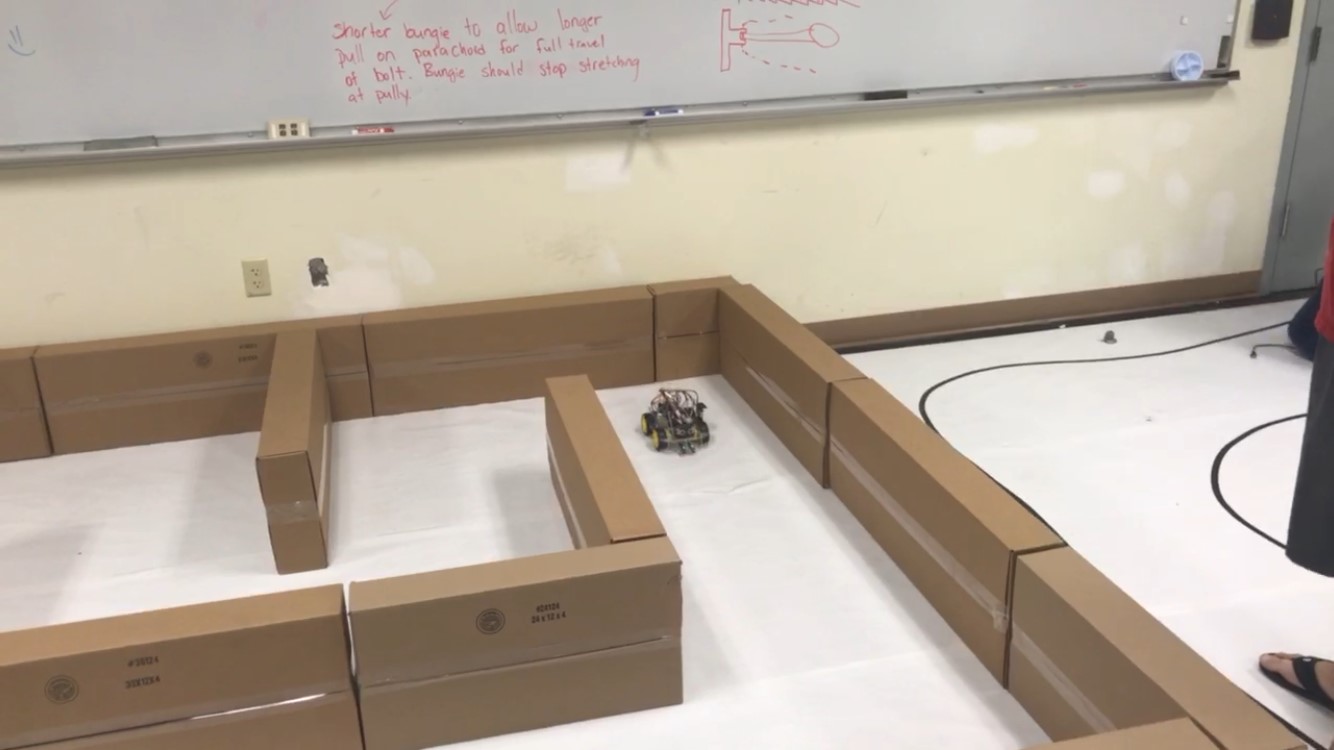
Arduino Sketch
//*****************************************LIBRARIES*************************************************
#include <Adafruit_MotorShield.h>
#include <encoder.h>
#include <motor.h>
#include <rangefinder.h>
#include <speedcontrol.h>
#include <Wire.h>
//*******************CREATE THE MOTOR SHIELD OBJECT WITH THE DEFAULT I2C ADDRESS*********************
Adafruit_MotorShield AFMS = Adafruit_MotorShield();
//*****************************GLOBAL VARIABLES ARE DECLARED HERE************************************
volatile float frontSensorDistance = 0.0;
volatile float leftSensorDistance = 0.0;
//**********************************POINTERS ARE DECLARED HERE***************************************
motor *RFMotor;
motor *LFMotor;
motor *LRMotor;
motor *RRMotor;
Range_Echo *frontRange;
Range_Echo *leftRange;
//===================================================================================================
//=========================================SETUP STARTS HERE=========================================
//===================================================================================================
void setup() {
//******************************************REGISTERS USED*******************************************
AFMS.begin();
frontSensorDistance = (frontRange -> Timing()) * UNIT_CONVERSION;
leftSensorDistance = (leftRange -> Timing()) * UNIT_CONVERSION;
RFMotor = new motor(&AFMS, 1, 0); //right front
LFMotor = new motor(&AFMS, 2, 1); //left front
LRMotor = new motor(&AFMS, 3, 1); //left rear
RRMotor = new motor(&AFMS, 4, 0); //right rear
frontRange = new Range_Echo(10, 11);
leftRange = new Range_Echo(8, 9);
}
//===================================================================================================
//==========================================LOOP STARTS HERE=========================================
//===================================================================================================
void loop() {
frontSensorDistance = (frontRange -> Timing()) * UNIT_CONVERSION;
leftSensorDistance = (leftRange -> Timing()) * UNIT_CONVERSION;
if ((frontSensorDistance > 8.0) && (leftSensorDistance > 17.0)) {
LFMotor->setDrive(90.0, FORWARD);
LRMotor->setDrive(90.0, FORWARD);
RFMotor->setDrive(94.0, FORWARD);
RRMotor->setDrive(94.0, FORWARD);
}
else if ((frontSensorDistance <= 7.0) && (frontSensorDistance > 1.0) && (leftSensorDistance <= 10.0)) { // RIGHT turn
for (int x = 0; x < 48; x++) {
LFMotor->setDrive(200.0, FORWARD);
LRMotor->setDrive(200.0, FORWARD);
RFMotor->setDrive(198.0, BACKWARD);
RRMotor->setDrive(198.0, BACKWARD);
}
}
else if ((frontSensorDistance <= 7.0) && (frontSensorDistance > 1.0) && (leftSensorDistance > 10.0)) { // LEFT turn
for (int x = 0; x < 48; x++) {
LFMotor->setDrive(200.0, BACKWARD);
LRMotor->setDrive(200.0, BACKWARD);
RFMotor->setDrive(200.0, FORWARD);
RRMotor->setDrive(200.0, FORWARD);
}
}
else {
LFMotor->setDrive(90.0, FORWARD);
LRMotor->setDrive(90.0, FORWARD);
RFMotor->setDrive(94.0, FORWARD);
RRMotor->setDrive(94.0, FORWARD);
}